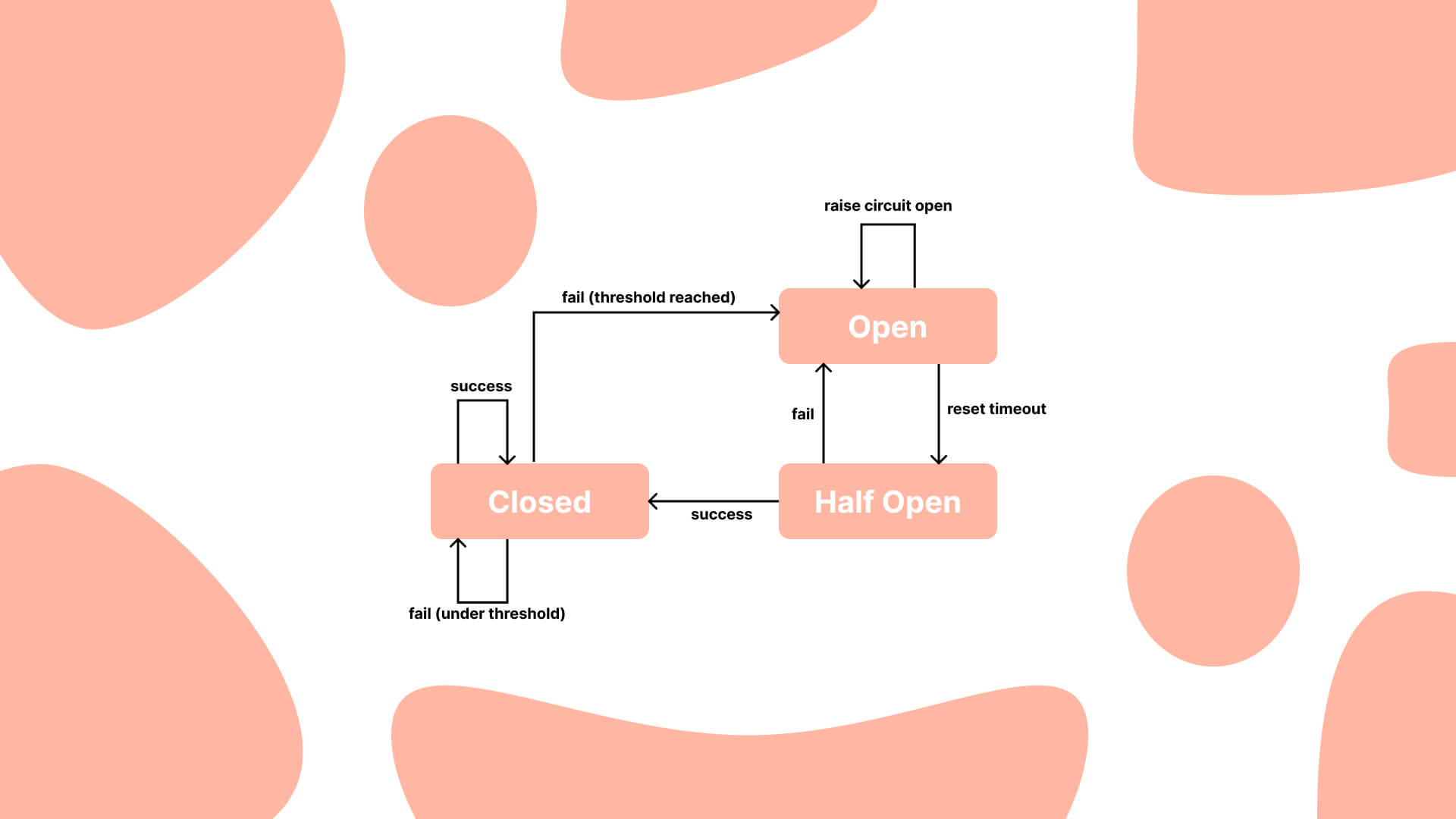
Have you ever heard of the Circuit Breaker pattern? It’s a design pattern that helps prevent failures in a distributed system by stopping a cascade of failures from happening. It’s a way of protecting your system from overloading and failing.
The Circuit Breaker pattern works by introducing a “circuit breaker” component that acts as a proxy between the calling service and the service that it’s calling. The circuit breaker keeps track of the health of the service it’s calling and, if it detects that the service is failing, it will open the circuit and stop sending requests to the failing service. This prevents the calling service from getting bogged down with failed requests and allows it to continue functioning.
Circuit Breaker Implementation
Here’s an example of how you might implement the Circuit Breaker pattern in Golang:
type CircuitBreaker struct {
requests chan struct{}
timeout time.Duration
}
func NewCircuitBreaker(timeout time.Duration) *CircuitBreaker {
return &CircuitBreaker{
requests: make(chan struct{}, 1),
timeout: timeout,
}
}
func (cb *CircuitBreaker) Call(fn func() error) error {
select {
case cb.requests <- struct{}{}:
default:
return fmt.Errorf("circuit breaker is open")
}
defer func() { <-cb.requests }()
done := make(chan error, 1)
go func() { done <- fn() }()
select {
case err := <-done:
return err
case <-time.After(cb.timeout):
return fmt.Errorf("request timed out")
}
}
You can use the Circuit Breaker like this:
cb := NewCircuitBreaker(time.Second)
err := cb.Call(func() error {
// call the service you want to protect
return nil
})
if err != nil {
// handle error
}
Circuit Breaker Golang open-source libraries
There are several open-source libraries that have implemented the Circuit Breaker pattern in Golang. Here are a few popular ones:
- Hystrix: This is a library from Netflix that provides a wide range of fault tolerance features, including Circuit Breaker. It’s widely used in microservices architectures and is a great choice if you need a comprehensive fault tolerance solution. Here’s an example of how you might use Hystrix in Golang:
func main() {
hystrix.ConfigureCommand("my_command", hystrix.CommandConfig{
Timeout: 1000,
MaxConcurrentRequests: 100,
ErrorPercentThreshold: 25,
})
hystrix.Go("my_command", myCommand)
}
func myCommand() error {
// call the service you want to protect
return nil
}
- Go-Hystrix: This is a Go port of the Hystrix library. It provides similar functionality as Hystrix but is written in pure Golang and does not require any additional dependencies. Here’s an example of how you might use Go-Hystrix:
func main() {
hystrix.ConfigureCommand("my_command", hystrix.CommandConfig{
Timeout: 1000,
MaxConcurrentRequests: 100,
ErrorPercentThreshold: 25,
})
hystrix.Go("my_command", myCommand)
}
func myCommand() error {
// call the service you want to protect
return nil
}
- Go-Circuit: This is a lightweight Circuit Breaker library that provides a simple, easy-to-use API. It’s a good choice if you just need basic Circuit Breaker functionality and don’t need all the bells and whistles of a more comprehensive library. Here’s an example of how you might use Go-Circuit:
cb := circuit.NewThresholdBreaker(10)
err := cb.Call(func() error {
// call the service you want to protect
return nil
})
if err != nil {
// handle error
}
By using these libraries you can save a lot of development time and focus on the features of your application and not on the implementation of the pattern.
Bonus: Istio Service Mesh Circuit Breaker implementation
The Circuit Breaker pattern can also be implemented in an Istio service mesh, which is a way of managing and routing traffic in a microservices architecture. Istio has a built-in Circuit Breaker feature that can be used to automatically open and close the circuit based on the health of the service.
Here’s an example of how you might configure a Circuit Breaker in Istio:
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: myservice
spec:
hosts:
- myservice.com
http:
- route:
- destination:
host: myservice
retries:
attempts: 3
perTryTimeout: 2s
retryOn: gateway-error,connect-failure,refused-stream
circuitBreaker:
maxConnections: 10
httpMaxRequests: 5
httpConsecutiveErrors: 3
httpDetectionInterval: 5s
This configuration sets up a Circuit Breaker for the “myservice” service that will open the circuit if there are more than 10 connections, more than 5 HTTP requests in a 5 second interval, or 3 consecutive HTTP errors.
Afterwords
That’s the Circuit Breaker pattern in a nutshell. It’s a great way to protect your system from cascading failures and keep it running smoothly.
Hope that helps! Let me know if you have any questions about the Circuit Breaker pattern or how to implement it in Golang or Istio. One thing to keep in mind is that the Circuit Breaker pattern is not a silver bullet and should be used in conjunction with other techniques like load balancing, timeouts, and retries.
Additionally, it’s important to monitor the state of the circuit breaker and analyze the metrics to make sure that it’s working as expected. This will help you understand the health of your services and identify any issues before they become critical.
The Circuit Breaker pattern is a powerful tool for managing the complexity of a distributed system and ensuring that it remains resilient in the face of failures. It’s a great addition to your toolbox of techniques for building robust, fault-tolerant systems.